
Elements of Mule Programming
Mule 3 provides three different constructs that can be used to build applications:
-
Services are the classic Mule way of organizing message flow. Each service consists of three sections:
-
Input, where messages are received
-
An optional component, where any sort of application logic can be applied to a message
-
An optional output, where the messages are sent to other services or transports.
-
-
Flows, which are new in Mule 3. A flow is a combination of message sources and message processors that doesn’t have as fixed a format as a service does. It can be as simple or as complex as required, and can include, for instance, processing by multiple components before any output is performed.
-
Configuration Patterns are also new in Mule 3. A configuration pattern is like a pre-built flow: the logic is already built into it, so that only some simple tailoring is needed to make it functional.
A Mule application can contain any or all of these.
Within flows, the streamlined architecture of Mule 3 allows Transformers, Filters, Components, Control Message Flow and other message processing artifacts to be used, and nested, freely as required. They all implement a common MessageProcessor interface and can be used interchangeably. Services also allow these building blocks to be used in some specific extension points.
Here is information about the different building blocks provided by Mule presented in categories.
Message Sources

A message source receives or generates new messages to be processed by Mule. Mule supports the following types of message sources:
-
Inbound Endpoints
-
Custom message sources
Inbound Endpoints
Inbound Endpoints receive new messages from a channel or resource by using a server socket, polling a remote socket or resource, or by registering a listener.
For information about available transports see Connecting Using Transports
For information about configuring endpoints see Configuring Endpoints
Polls
Rather than using an inbound endpoint you can poll any message processor and use the result as the source of your flow. A frequency in milliseconds can be configured otherwise the default of 1s is used. Examples of things you can poll are outbound-endpoints, other flows or processor chains or any message processor.
To configure polling use the <poll> element instead of an inbound endpoint.
<flow name="myFlow">
<poll frequency="2000">
<http:outbound-endpoint host=".." port=".."/>
</poll>
<processor ref=""/>
<processor ref=""/>
</flow>
Currently <poll> has no support for cron expression of for customizing it’s threading profile
Custom Message Sources
You can replace any inbound endpoint in a flow with a custom message source. This allows you to use any class as a message source to the flow, including cloud connectors. You configure the custom message source using the <custom-source> element. In the element you identify the class for the custom source. You can further configure the custom message source using Spring bean properties.
The following code example configures a custom message source for a flow:
<flow name="useMyCustomSource">
<custom-source class="org.my.customClass">
<spring:property name="threads" value="500"/>
</custom-source>
<vm:outbound-endpoint path="output" exchange-pattern="one-way"/>
</flow>
Perform Some Logic

You’ll often need to perform some business logic as part of your flow. Mule supports components implemented in Java and using scripting languages.
Components can do whatever you need them to do including mutating the message if required. Components support the concept of entry point resolution and lifecycle adaption designed to accommodate the use of component implementations with Mule without modification.
Look here for more about configuring Mule Components.
Transform the Message

When external systems use different message formats or you need to convert the payload type you’ll need to one or more Message Transformers.
See Using Transformers for a list of available transformers as well as information on how to implement your own.
Filter messages
To only allow certain messages to continue to be processed you can use a Filter. Filters may filter based on message type, message contents or some other criteria. |
|
To prevent messages from being processed if security credentials are not provided or do not match you can use Security Filter |
|
To filer out duplicate messages you should use an Idempotent Filter |
Control Message Flow
There are a number of ways in which you can control message flow, which are described below. These are specified somewhat different in flows and services. In services, since the input and output sections are quite distinct, there are separate groups of Inbound Routers and Outbound Routers. In flows, routers are a subset of message processors.
Split or Aggregate Messages
![]() |
Message splitters allow a single incoming message to be split into n pieces each of the parts being passed onto the next message processor as a new message. |
---|---|
Aggregators do the opposite and aggregate multiple inbound messages into a single message. |
For information on provided splitter and aggregator implementations and details on how to implement your own see Message Splitting and Aggregation
Route Messages
![]() |
![]() |
---|
In order to determine message flow in runtime Message Routers are used. Message routing can be configured statically or is determined in runtime using message type, payload or properties or some other criteria. Some message routers route to a single route whereas other routers route to multiple routes.
Send Messages over a transport
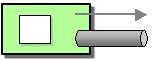
Once you have have completed message processing you may wish to send the resulting message to an external service or location. You may also need to invoke a remote service elsewhere in the flow.
Outbound endpoints are used to send messages over a channel using a transport.
For information about available transports see Connecting Using Transports
For information about configuring endpoints see Configuring Endpoints
Other
Message Processor Chain
A Message Processor Chain allows to define a reusable set of message processors that are chained together and invoked in sequence. When configuring Mule using XML a processor chain is defined using the processor-chain element.
<processor-chain name="myReusableChain">
<bytearray-to-object-transformer />
<expression-filter expression=""/>
<custom-processor class=""/>
</processor-chain>
Response Adaptor
A response adaptor is configured using the response element. It is used when you want to use a Message Processor on a response message. In the following case the append-string-transformer is invoked after response was received from the outbound endpoint invocation. This can be useful when you have a message process that performs response processing (e.g. CXF) and you need to add a message processor after this.
<http:outbound-endpoint address="http://foo.bar/formAction" exchange-pattern="request-response" method="POST">
<response>
<append-string-transformer message=" - RECEIVED BY MULE"/>
</response>
</http:outbound-endpoint>
In the following example response block is invoked after the flow finished processing and before the response message is returned to the caller of the inbound endpoint.
<flow ...>
<http:inbound-endpoint address="http://localhost:8080/hello" exchange-pattern="request-response">
<response>
<message-properties-transformer>
<add-message-property key="Content-Type" value="text/html"/>
</message-properties-transformer>
</response>
</http:inbound-endpoint>
<component class="com.foo.Bar"/>
</flow>
Custom Message Processors
Custom Message Processors can be implemented by simply extending the MessageProcessor or InterceptingMessageProcessor interface and using the <custom-processor> element. If you prefer to used a referenced spring bean as a message processor then you can use the standard <processor ref=""/> element and reference it directly.
Configuring a custom message processor with a class name
<custom-processor name="customMsgProc" class=""/>
Configuring a custom message processor by referencing a spring bean
<processor ref="myBean" />
For information on implementing your own Filters or Transformers see the respective pages. There is also more detailed information on implementing your own Custom Message Processors.