STUDIO Visual Editor
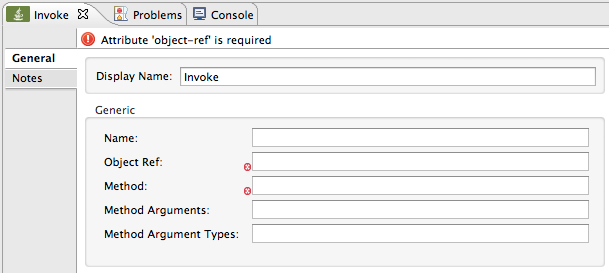
Field | Description | Required? | Example XML |
---|---|---|---|
Display Name |
Customize to display a unique name for the component in your application. |
|
|
Name |
The name of the message processor for logging purposes. |
|
|
Object Ref |
Reference to the object containing the method to be invoked. |
X |
|
Method |
The name of the method to be invoked. |
X |
|
Method Arguments |
Comma-separated list of Mule expressions that, when evaluated, are the arguments for the method invocation. |
|
|
Method Argument Types |
Comma-separated list of argument types for the method invocation. Use when you have more than one method with the same name in your class. |
`methodArgumentTypes="java.lang.Float, java.lang.Float" ` |
<spring:beans>
<spring:bean name="beanName" class="invoke.ClassName"/>
</spring:beans>
<flow
...
<invoke object-ref="beanName" method="addTwoNumbers" methodArguments="#[1], #[2]" methodArgumentTypes="java.lang.Float, java.lang.Float" name="someName" doc:name="Invoke"/>
...
</flow>
XML Editor or Standalone
Include the invoke method in your flow, and define a Spring bean as a global element with the reference to the object containing the method.
Element | Description |
---|---|
|
Invokes a method in a specified object using method arguments determined by Mule expressions. |
Attributes:
Attribute | Description | Required? |
---|---|---|
|
Customize to display a unique name for the component in your application. Not required for Mule Standalone. |
|
|
The name of the message processor for logging purposes. |
|
|
Reference to the object containing the method to be invoked. |
X |
|
The name of the method to be invoked. |
X |
|
Comma-separated list of Mule expressions that, when evaluated, provide the arguments for the method invocation. |
|
|
Comma-separated list of argument types for the method invocation. Use when you have more than one method with the same name in your class. |
<spring:beans>
<spring:bean name="beanName" class="invoke.ClassName"/>
</spring:beans>
<flow
...
<invoke object-ref="beanName" method="addTwoNumbers" methodArguments="#[1], #[2]" methodArgumentTypes="java.lang.Float, java.lang.Float" name="someName" doc:name="Invoke"/>
...
</flow>